Threw the muzzle flash 3d model into the explosion so we get some kinda fire out of it. It works much better than I expected:
Also attempted to make some light-bulbs that hang from the ceiling but it turns out the unreal provided cable component (https://dev.epicgames.com/documentation/en-us/unreal-engine/cable-components-in-unreal-engine) doesn’t apply physics forces to whatever it’s attached to.
The red line is a line trace I perform upwards to find any actors that are willing to take the lightbulb:
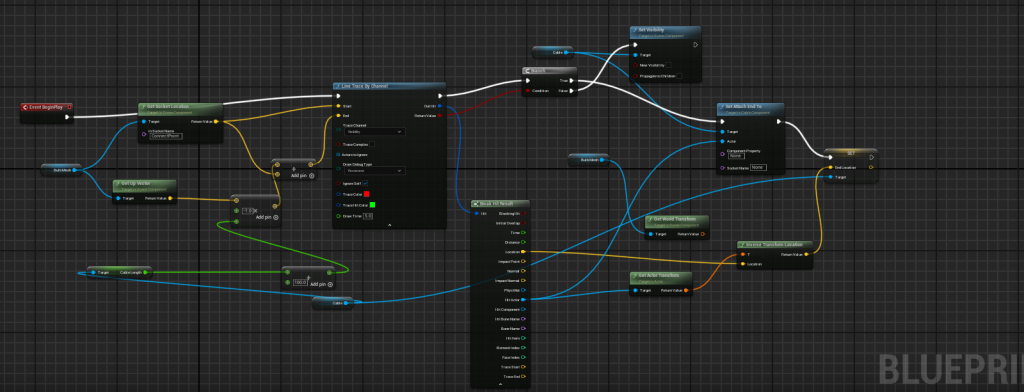
So I’ll have to essentially make a bone heavy mesh and attach that to the lightbulb.
Also the first level is progressing:
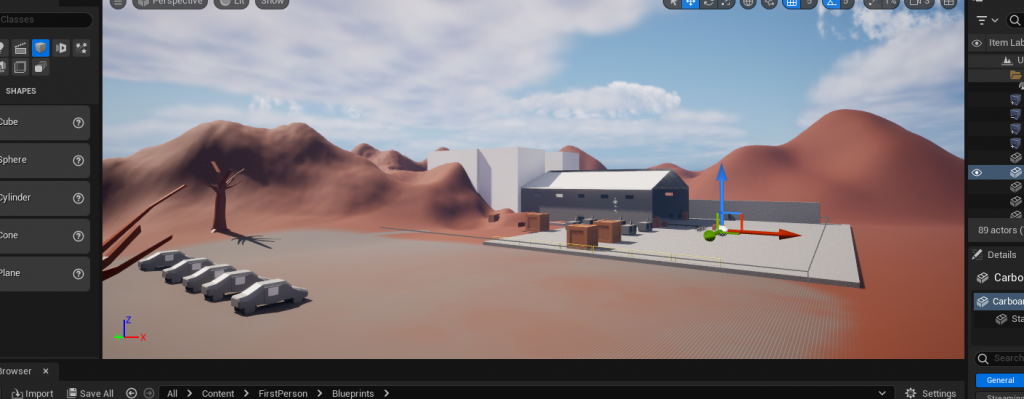
Slow pace but I think I should have something within a week. Going with the desert motif so I don’t have to make too many buildings..
Also I made an alert sound for the bots:
I made the sound in Abelton but I didn’t save the set….Essentially it was an Up only arpeggiator into an operator preset into a redux into a compress and eq-8. Also “arpeggiator” I found out isn’t in the dictionary:
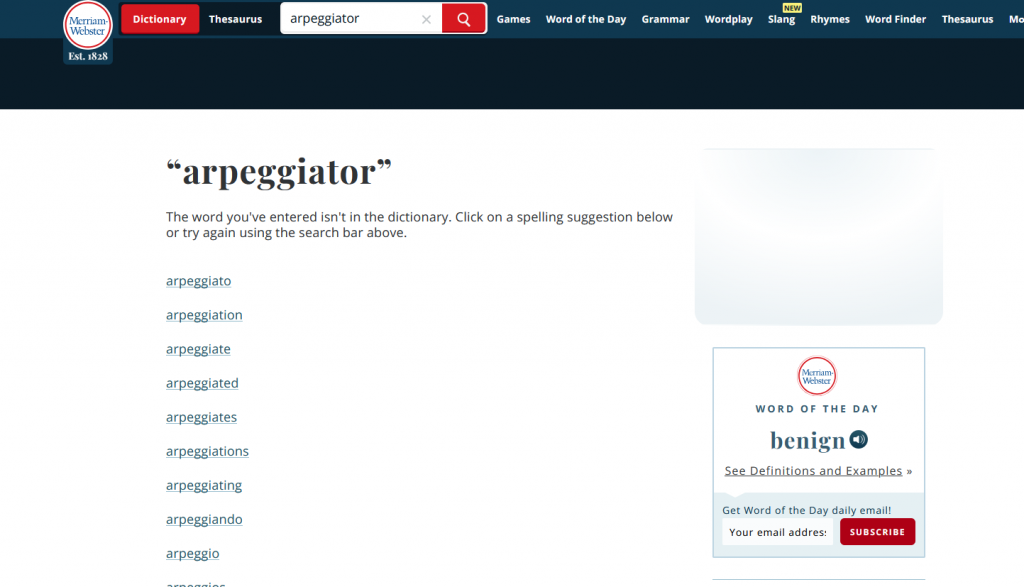
Which: https://www.sweetwater.com/store/search?s=arpeggiator#search-header (P.S. VERY overpriced for what you can achieve with a $20 arduino uno and a spliced midi cable: https://github.com/FortySevenEffects/arduino_midi_library ).