I wanted to mesh paint (https://dev.epicgames.com/documentation/en-us/unreal-engine/getting-started-with-mesh-texture-color-painting-in-unreal-engine) a bit in unreal and thought “Oh I’ll just flip this virutal texture box”
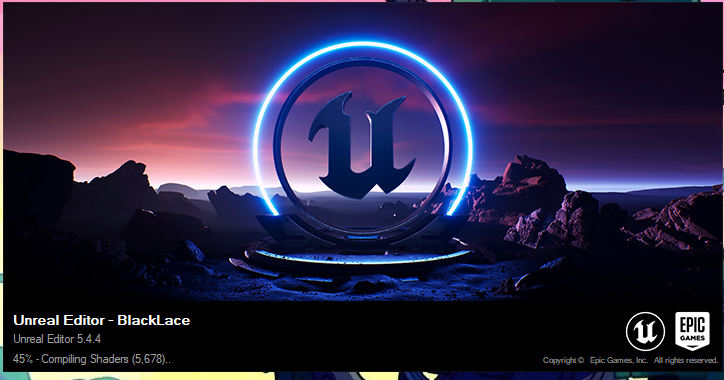
I have a pretty good pc (4090 24gb) I’m surprised its taking so 5+ minutes (but then again I’ve made NO effort to optimize any of my materials…
That being said…
I guess I made things low poly enough that maybe this is inherently optimized….
But thats a detail thing that I can work on later, For the outside I want to:
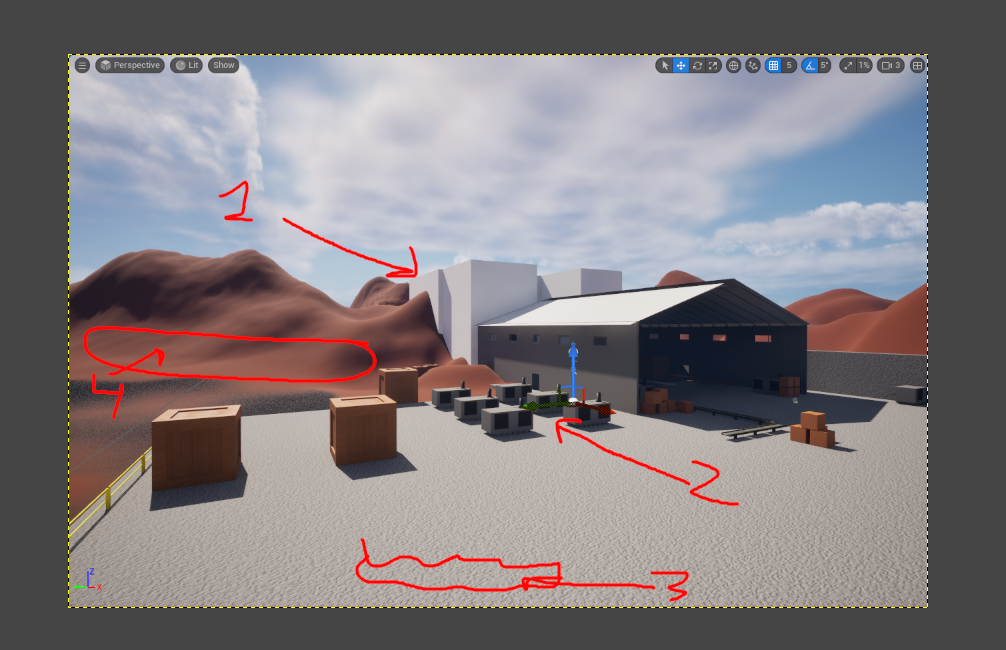
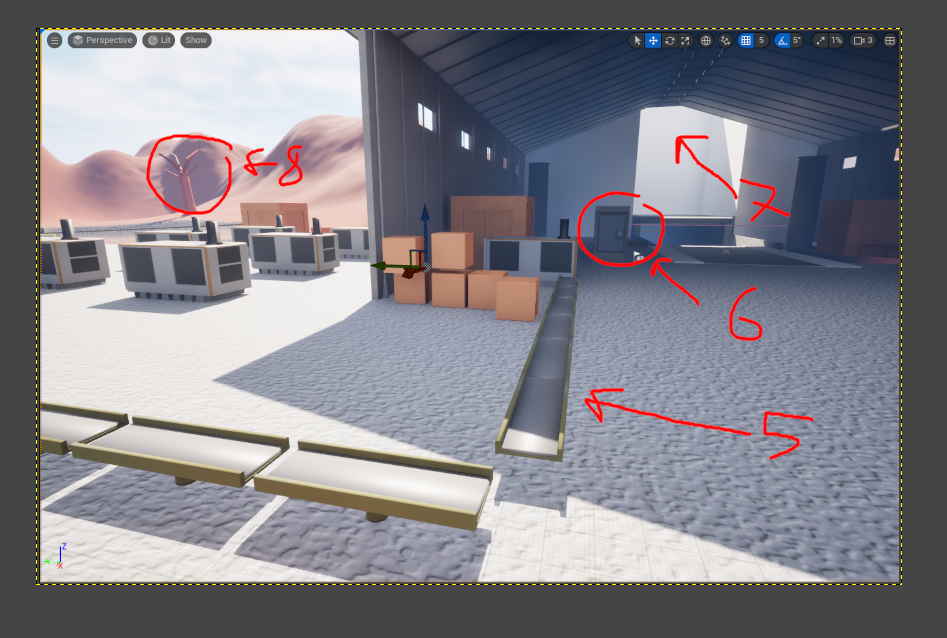
- Make those back blocks look like a office building embedded into the rock
- Replace those generators with something that would flow better with game play
- Make a new texture for that platform. Tat texture is fine but I’m using it in like 12 places and I don’t want to mess with UV scaling just to get it working
- Make some static rock models to place along the edges of mountainous regions so I it doesn’t look like a muddy ski slope
- Do something better with these conveyor belts. I put in WAAY to much time to make those kinda work, I might just delete them
- I have this cabinet which is kinda sketchy looking, it was supposed to go with a terminal but I might move it again
- Fix that lighting so that there isn’t a weird skylight for a warehouse setting
- Get some friends for that tree.
Also I need to make a better road texture, at far distances it looks fine but then you zoom in…
Every-time I look at it my graphics card fan spins up…probably a bad thing.
I also added a tunnel model
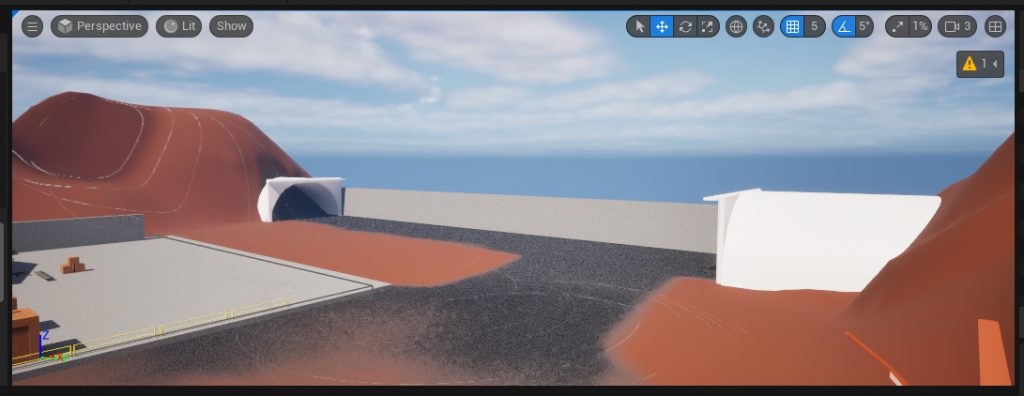
The UV’s and normals are REALLY messed up atm but I dont want to dig in yet. I fixed it by making the underlying material double sided but generally if you’re a single sided material and your model goes from looking like this in blender:
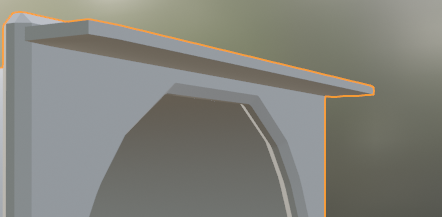
To this…
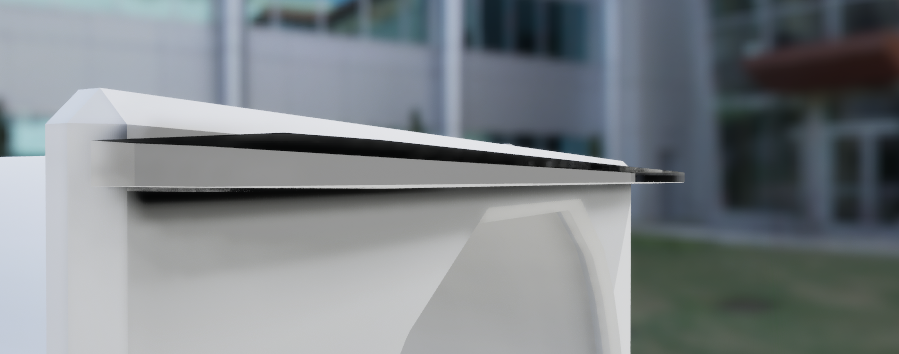
You should probably do it again….
But in the meantime there’s the magic “Make the material two sided” button which is normally used for meshes that have both an interior and exterior (which is not this….at all)
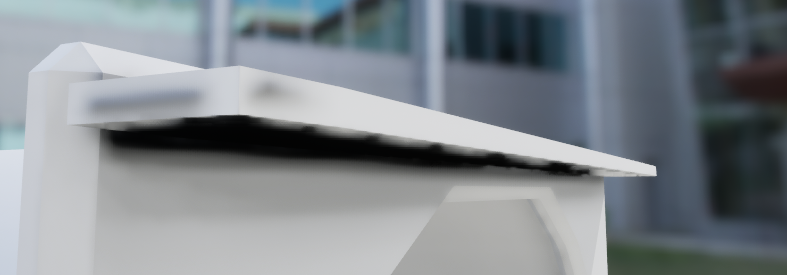
Still has issues. The darkness underneath comes from the unreal seeing that the normal of the face is facing in a direction that is into the block’s origin rather than away from it. This makes the lighting calculations get all wonky.
I’ve been disorganized when working this (also I stopped working it for like 2 weeks and now I’m lost) So I made a trello board here:
https://trello.com/b/dmIooAod/blacklaceworking-board
Broken into:
“Longer term goals”- Things I probably wont look into until I have something on steam
“MVP Make/Design”- Things I want to add to the game before I submit a demo/game to steamworks
“Fix/Improve” – Things that are in the game but need to be re-worked.
I’ll start chipping away at these and my personal goal is to get something ready to throw on steam by June.